Serv00上搭建一个 PixPro 若梦图床 + 接入Cloudflare R2
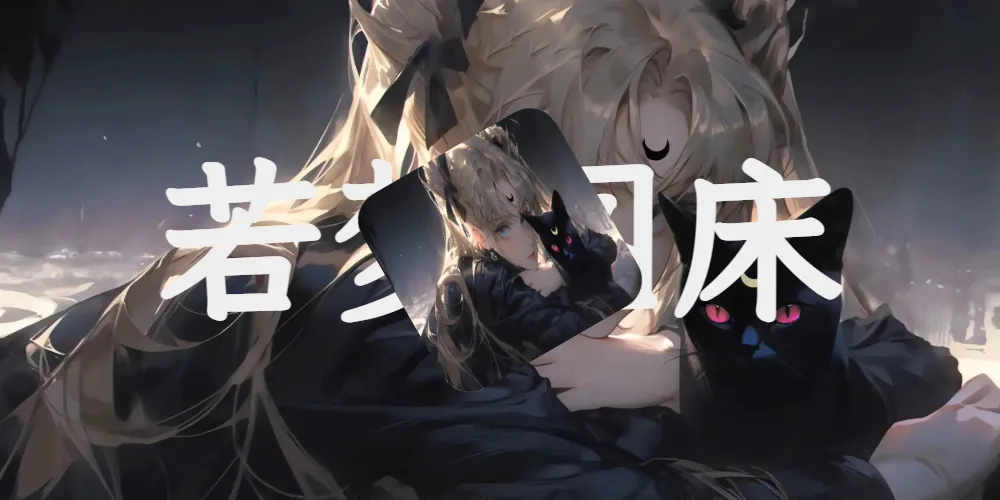
AI-摘要
Jinx GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
Serv00上搭建一个 PixPro 若梦图床 + 接入Cloudflare R2
东方月初Serv00上搭建一个PixPro图床,内存太小,我们可以接入到CFR2 足够个人使用。自己有VPS的也可在宝塔上面部署
面板页
后台页
安装前准备
安装步骤
- 注册好Serv00账号打开网页进入到面板端添加域名或者用自己的域名都行,这里我用别的网站注册的二级域名。如下图添加
- 把里面的A记录解析你托管到CF的域名或者别的地方申请的
- 按照下图打开域名的权限
点击Save等待成功
- 创建MySQL数据库(保存账号及地址)
上传安装包并解压进入文件管理器
- 进入public_html文件夹删除其下所有文件
- 上传安装包到你添加的域名或者Serv00自带域名下
- 选择你们下载文件的路径上传好如下图
和上一步一样
- 进去后如下图所以我们
Shift
建一直按着然后点击鼠标左键全选
- 移动到我们开始创建域名目录下的
public_html
- 修改PHP版本 在域名目录下创建一个文本,名为:
.htaccess
- 添加PHP版本
选择Text Editor
添加以下代码
1 | AddType application/x-httpd-php81 .php |
- 访问你的域名开始安装系统,管理员账户和密码自己设置 ,如果要接入
CFR2
我们存储选择S3
下面我会写S3
安装方式
- 点击回到首页就可以上传图片了
接入R2 前提 已开通 Cloudflare R2
进入到 Cloudflare 面板 找到 R2 右边有一个管理 R2API 令牌点击创建 API令牌 ,创建好后里面有我们需要的访问密钥和机密访问密钥
1. 进入到 Cloudflare 面板 找到 R2 点击创建存储桶
2. 进入后名称随便写。位置选择亚太地区后点击创建存储桶
3. 创建好后点击设置拉到下面看到 R2.dev 子域点击后面的允许访问 , 连接域需要添加你托管到CF上面域名
4. 14 步我们安装选择S3
后看到下图
参数 | 值 |
---|---|
S3 Region | auto |
S3 Bucket | 你的存储桶名称 |
S3 Endpoint | 你的S3API 后面不要加你的存储桶名称 |
S3 Access Key ID | R2API令牌访问密钥 |
S3 Access Key Secret | R2API令牌机密访问密钥 |
S3 自定义域名 | R2.dev 添加的自定义域 或 R2 分配的域名 |
5. 点击完成安装即可。这是我们上传好的图片 我下面的域名就是我们 R2 分配的域名
6. 我们回到 Cloudflare R2 就会看到刚刚上传的图片
在给大家推荐一个的在线生成封面网站,专为博客、短视频、社交媒体等生成个性化封面.GitHub,项目地址.这个封面可以和图床结合。 封面做好后我们不用去上传到图床直接点击封面网站的外链即可,前提已部署
PixPro
图床
感谢作者梦爱吃鱼,欢迎大家去预览、哈哈哈
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果